Loading [MathJax]/extensions/tex2jax.js
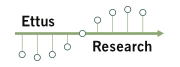 |
USRP Hardware Driver and USRP Manual
Version: 4.8.0.0
UHD and USRP Manual
|
|
Go to the documentation of this file.
18 struct uhd_sensor_value_t
21 std::unique_ptr<uhd::sensor_value_t> sensor_value_cpp;
22 std::string last_error;
26 struct uhd_sensor_value_t;
85 const char* formatter);
100 const char* formatter);
UHD_API uhd_error uhd_sensor_value_to_int(uhd_sensor_value_handle h, int *value_out)
Get the sensor's value as an integer.
UHD_API uhd_error uhd_sensor_value_make_from_int(uhd_sensor_value_handle *h, const char *name, int value, const char *unit, const char *formatter)
Make a UHD sensor from an integer.
UHD_API uhd_error uhd_sensor_value_to_realnum(uhd_sensor_value_handle h, double *value_out)
Get the sensor's value as a real number.
@ UHD_SENSOR_VALUE_STRING
Definition: sensors.h:42
UHD_API uhd_error uhd_sensor_value_free(uhd_sensor_value_handle *h)
Free the given sensor handle.
uhd_error
UHD error codes.
Definition: error.h:20
@ UHD_SENSOR_VALUE_INTEGER
Definition: sensors.h:40
UHD_API uhd_error uhd_sensor_value_value(uhd_sensor_value_handle h, char *value_out, size_t strbuffer_len)
Get the sensor's value.
UHD_API uhd_error uhd_sensor_value_unit(uhd_sensor_value_handle h, char *unit_out, size_t strbuffer_len)
Get the sensor's unit.
#define UHD_API
Definition: config.h:87
UHD_API uhd_error uhd_sensor_value_make_from_string(uhd_sensor_value_handle *h, const char *name, const char *value, const char *unit)
Make a UHD sensor from a string.
uhd_sensor_value_data_type_t
Sensor value types.
Definition: sensors.h:38
UHD_API uhd_error uhd_sensor_value_data_type(uhd_sensor_value_handle h, uhd_sensor_value_data_type_t *data_type_out)
UHD_API uhd_error uhd_sensor_value_make_from_bool(uhd_sensor_value_handle *h, const char *name, bool value, const char *utrue, const char *ufalse)
Make a UHD sensor from a boolean.
UHD_API uhd_error uhd_sensor_value_make(uhd_sensor_value_handle *h)
Make an empty UHD sensor value.
@ UHD_SENSOR_VALUE_REALNUM
Definition: sensors.h:41
UHD_API uhd_error uhd_sensor_value_to_pp_string(uhd_sensor_value_handle h, char *pp_string_out, size_t strbuffer_len)
Get a pretty-print representation of the given sensor.
UHD_API uhd_error uhd_sensor_value_to_bool(uhd_sensor_value_handle h, bool *value_out)
Get the sensor's value as a boolean.
UHD_API uhd_error uhd_sensor_value_make_from_realnum(uhd_sensor_value_handle *h, const char *name, double value, const char *unit, const char *formatter)
Make a UHD sensor from a real number.
struct uhd_sensor_value_t * uhd_sensor_value_handle
C-level interface for a UHD sensor.
Definition: sensors.h:35
UHD_API uhd_error uhd_sensor_value_name(uhd_sensor_value_handle h, char *name_out, size_t strbuffer_len)
Get the sensor's name.
UHD_API uhd_error uhd_sensor_value_last_error(uhd_sensor_value_handle h, char *error_out, size_t strbuffer_len)
Get the last error logged by the sensor handle.
@ UHD_SENSOR_VALUE_BOOLEAN
Definition: sensors.h:39