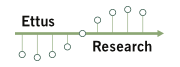 |
USRP Hardware Driver and USRP Manual
Version: 4.4.0.HEAD-0-g5fac246b
UHD and USRP Manual
|
|
Go to the documentation of this file.
16 #include <boost/core/demangle.hpp>
25 namespace uhd {
namespace experts {
56 virtual const std::string&
get_dtype()
const = 0;
58 virtual std::string
to_string()
const = 0;
78 const std::string _name;
85 template <
typename data_t>
86 static std::string
print(
const data_t& val)
88 std::ostringstream os;
93 static std::string
print(
const uint8_t& val)
95 std::ostringstream os;
102 std::ostringstream os;
126 template <
typename data_t>
135 data_node_t(
const std::string& name, std::recursive_mutex* mutex = NULL)
137 , _callback_mutex(mutex)
144 const std::string& name,
const data_t& value, std::recursive_mutex* mutex = NULL)
146 , _callback_mutex(mutex)
155 static const std::string dtype(boost::core::demangle(
typeid(data_t).name()));
172 return _data.is_dirty();
186 void set(
const data_t& value)
200 if (_callback_mutex == NULL)
202 "node " +
get_name() +
" is missing the callback mutex");
203 std::lock_guard<std::recursive_mutex> lock(*_callback_mutex);
206 if (
is_dirty() and has_write_callback()) {
214 if (_callback_mutex == NULL)
216 "node " +
get_name() +
" is missing the callback mutex");
217 std::lock_guard<std::recursive_mutex> lock(*_callback_mutex);
218 if (has_read_callback()) {
219 _rd_callback(std::string(
get_name()));
231 bool has_write_callback()
const override
233 return bool(_wr_callback);
236 void clear_write_callback()
override
238 _wr_callback =
nullptr;
246 bool has_read_callback()
const override
248 return bool(_rd_callback);
251 void clear_read_callback()
override
253 _rd_callback =
nullptr;
256 std::recursive_mutex* _callback_mutex;
259 dirty_tracked<data_t> _data;
279 virtual dag_vertex_t& retrieve(
const std::string& name)
const = 0;
306 template <
typename data_t>
345 + boost::core::demangle(
typeid(data_t).name())
346 +
" but got " + node().get_dtype());
367 template <
typename data_t>
376 inline const data_t&
get()
const
381 inline operator const data_t&()
const
393 return !(
get() == rhs);
410 template <
typename data_t>
419 inline const data_t&
get()
const
424 inline operator const data_t&()
const
436 return !(
get() == rhs);
439 inline void set(
const data_t& value)
475 std::list<std::string> retval;
477 retval.push_back(acc->node().get_name());
484 std::list<std::string> retval;
486 retval.push_back(acc->node().get_name());
499 _inputs.push_back(&accessor);
501 _outputs.push_back(&accessor);
509 bool is_dirty()
const override
511 bool inputs_dirty =
false;
513 inputs_dirty |= acc->node().is_dirty();
518 void mark_clean()
override
520 for (data_accessor_t* acc : _inputs) {
521 acc->node().mark_clean();
525 void resolve()
override = 0;
528 const std::string& get_dtype()
const override
530 static const std::string dtype =
"<worker>";
534 std::string to_string()
const override
541 bool has_write_callback()
const override
545 void clear_write_callback()
override {}
547 bool has_read_callback()
const override
551 void clear_read_callback()
override {}
553 std::list<data_accessor_t*> _inputs;
554 std::list<data_accessor_t*> _outputs;
@ ACCESS_READER
Definition: expert_nodes.hpp:28
dag_vertex_t & _vertex
Definition: expert_nodes.hpp:303
virtual void mark_clean()=0
bool operator!=(const data_t &rhs)
Definition: expert_nodes.hpp:434
data_accessor_base(const node_retriever_t &r, const std::string &n, const node_access_t a)
Definition: expert_nodes.hpp:338
virtual void clear_write_callback()=0
virtual void set_write_callback(const callback_func_t &func)=0
void set(const data_t &value)
Definition: expert_nodes.hpp:439
virtual std::string to_string() const =0
data_node_t(const std::string &name, std::recursive_mutex *mutex=NULL)
Definition: expert_nodes.hpp:135
std::list< std::string > get_inputs() const
Definition: expert_nodes.hpp:473
const data_t & get() const
Definition: expert_nodes.hpp:419
node_class_t
Definition: expert_nodes.hpp:27
const std::string & get_name() const
Definition: expert_nodes.hpp:51
virtual bool is_dirty() const =0
void bind_accessor(data_accessor_t &accessor)
Definition: expert_nodes.hpp:496
const data_t & get() const
Definition: expert_nodes.hpp:192
Definition: expert_nodes.hpp:368
Definition: expert_nodes.hpp:467
node_author_t get_author() const
Definition: expert_nodes.hpp:164
data_reader_t(const node_retriever_t &retriever, const std::string &node)
Definition: expert_nodes.hpp:371
bool is_dirty() const override
Definition: expert_nodes.hpp:170
void mark_clean() override
Definition: expert_nodes.hpp:175
@ CLASS_PROPERTY
Definition: expert_nodes.hpp:27
@ AUTHOR_EXPERT
Definition: expert_nodes.hpp:29
void set(const data_t &value)
Definition: expert_nodes.hpp:186
double get_real_secs(void) const
data_node_t(const std::string &name, const data_t &value, std::recursive_mutex *mutex=NULL)
Definition: expert_nodes.hpp:143
data_writer_t< data_t > & operator=(const data_writer_t< data_t > &value)
Definition: expert_nodes.hpp:450
static std::string print(const uint8_t &val)
Definition: expert_nodes.hpp:93
const std::string & get_dtype() const override
Definition: expert_nodes.hpp:153
@ AUTHOR_NONE
Definition: expert_nodes.hpp:29
node_author_t get_author() const
Definition: expert_nodes.hpp:332
bool is_reader() const override
Definition: expert_nodes.hpp:312
const node_access_t _access
Definition: expert_nodes.hpp:351
virtual const std::string & get_dtype() const =0
std::string to_string() const override
Definition: expert_nodes.hpp:159
node_class_t get_class() const
Definition: expert_nodes.hpp:46
bool is_writer() const override
Definition: expert_nodes.hpp:317
bool operator==(const data_t &rhs)
Definition: expert_nodes.hpp:386
~data_accessor_base() override
Definition: expert_nodes.hpp:310
bool is_dirty() const
Definition: expert_nodes.hpp:322
virtual const dag_vertex_t & lookup(const std::string &name) const =0
static std::string print(const data_t &val)
Definition: expert_nodes.hpp:86
virtual ~node_retriever_t()
Definition: expert_nodes.hpp:274
virtual bool is_reader() const =0
virtual ~data_accessor_t()
Definition: expert_nodes.hpp:292
virtual void set_read_callback(const callback_func_t &func)=0
virtual bool has_write_callback() const =0
@ AUTHOR_USER
Definition: expert_nodes.hpp:29
node_class_t get_class() const
Definition: expert_nodes.hpp:327
virtual dag_vertex_t & node() const =0
Definition: build_info.hpp:12
Definition: time_spec.hpp:28
const data_t retrieve() const
Definition: expert_nodes.hpp:212
Definition: expert_nodes.hpp:127
bool operator!=(const data_t &rhs)
Definition: expert_nodes.hpp:391
data_accessor_t(const node_retriever_t &r, const std::string &n)
Definition: expert_nodes.hpp:299
std::list< std::string > get_outputs() const
Definition: expert_nodes.hpp:482
virtual bool has_read_callback() const =0
worker_node_t(const std::string &name)
Definition: expert_nodes.hpp:470
void commit(const data_t &value)
Definition: expert_nodes.hpp:198
node_access_t
Definition: expert_nodes.hpp:28
Definition: expert_nodes.hpp:271
Definition: exception.hpp:95
void resolve() override
Definition: expert_nodes.hpp:180
Definition: expert_nodes.hpp:289
const data_t & get() const
Definition: expert_nodes.hpp:376
friend std::ostream & operator<<(std::ostream &os, const data_reader_t &reader)
Definition: expert_nodes.hpp:396
@ CLASS_DATA
Definition: expert_nodes.hpp:27
node_author_t
Definition: expert_nodes.hpp:29
data_writer_t(const node_retriever_t &retriever, const std::string &node)
Definition: expert_nodes.hpp:414
static std::string print(const time_spec_t time)
Definition: expert_nodes.hpp:100
virtual ~dag_vertex_t()
Definition: expert_nodes.hpp:43
Definition: exception.hpp:47
std::function< void(std::string)> callback_func_t
Definition: expert_nodes.hpp:41
Definition: expert_nodes.hpp:411
Definition: expert_nodes.hpp:38
boost::noncopyable noncopyable
Definition: noncopyable.hpp:45
Definition: expert_nodes.hpp:307
virtual void clear_read_callback()=0
@ CLASS_WORKER
Definition: expert_nodes.hpp:27
@ ACCESS_WRITER
Definition: expert_nodes.hpp:28
bool operator==(const data_t &rhs)
Definition: expert_nodes.hpp:429
data_writer_t< data_t > & operator=(const data_t &value)
Definition: expert_nodes.hpp:444
virtual bool is_writer() const =0
Definition: expert_nodes.hpp:81
data_node_t< data_t > * _datanode
Definition: expert_nodes.hpp:350
dag_vertex_t(const node_class_t c, const std::string &n)
Definition: expert_nodes.hpp:74