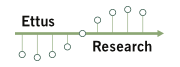 |
USRP Hardware Driver and USRP Manual
Version: 4.4.0.HEAD-0-g5fac246b
UHD and USRP Manual
|
|
Go to the documentation of this file.
27 struct uhd_subdev_spec_t {
29 std::string last_error;
34 struct uhd_subdev_spec_t;
120 UHD_API void uhd_subdev_spec_pair_cpp_to_c(
UHD_API uhd_error uhd_subdev_spec_last_error(uhd_subdev_spec_handle h, char *error_out, size_t strbuffer_len)
Get the last error recorded by the given handle.
UHD_API uhd_error uhd_subdev_spec_free(uhd_subdev_spec_handle *h)
Safely destroy a subdevice specification handle.
struct uhd_subdev_spec_t * uhd_subdev_spec_handle
A C-level interface for working with a list of subdevice specifications.
Definition: subdev_spec.h:44
uhd_error
UHD error codes.
Definition: error.h:20
Subdevice specification.
Definition: subdev_spec.h:16
#define UHD_API
Definition: config.h:87
UHD_API uhd_error uhd_subdev_spec_pairs_equal(const uhd_subdev_spec_pair_t *first, const uhd_subdev_spec_pair_t *second, bool *result_out)
Check to see if two subdevice specifications are equal.
UHD_API uhd_error uhd_subdev_spec_pair_free(uhd_subdev_spec_pair_t *subdev_spec_pair)
Safely destroy any memory created in the generation of a uhd_subdev_spec_pair_t.
Definition: subdev_spec.hpp:20
UHD_API uhd_error uhd_subdev_spec_to_pp_string(uhd_subdev_spec_handle h, char *pp_string_out, size_t strbuffer_len)
Get a string representation of the given list.
char * sd_name
Subdevice name.
Definition: subdev_spec.h:20
UHD_API uhd_error uhd_subdev_spec_push_back(uhd_subdev_spec_handle h, const char *markup)
Add a subdevice specification to this list.
UHD_API uhd_error uhd_subdev_spec_make(uhd_subdev_spec_handle *h, const char *markup)
Create a handle for a list of subdevice specifications.
UHD_API uhd_error uhd_subdev_spec_at(uhd_subdev_spec_handle h, size_t num, uhd_subdev_spec_pair_t *subdev_spec_pair_out)
Get the subdevice specification at the given index.
char * db_name
Definition: subdev_spec.h:18
Definition: subdev_spec.hpp:59
UHD_API uhd_error uhd_subdev_spec_size(uhd_subdev_spec_handle h, size_t *size_out)
Check how many subdevice specifications are in this list.
UHD_API uhd_error uhd_subdev_spec_to_string(uhd_subdev_spec_handle h, char *string_out, size_t strbuffer_len)
Get a markup string representation of the given list.