Loading [MathJax]/extensions/tex2jax.js
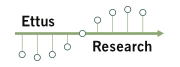 |
USRP Hardware Driver and USRP Manual
Version: 4.8.0.0
UHD and USRP Manual
|
|
Go to the documentation of this file.
40 using sptr = std::shared_ptr<extension>;
45 virtual std::string get_name() = 0;
47 static void register_extension(
60 #define UHD_REGISTER_EXTENSION(NAME, CLASS_NAME) \
61 UHD_STATIC_BLOCK(register_extension_##NAME) \
63 uhd::extension::extension::register_extension(#NAME, CLASS_NAME::make); \
std::function< sptr(factory_args)> factory_type
Definition: extension.hpp:41
std::shared_ptr< extension > sptr
Definition: extension.hpp:40
#define UHD_API
Definition: config.h:87
uhd::rfnoc::radio_control::sptr radio_ctrl
Definition: extension.hpp:36
uhd::rfnoc::mb_controller::sptr mb_ctrl
Definition: extension.hpp:37
Definition: extension.hpp:26
Definition: extension.hpp:34
Definition: core_iface.hpp:25
std::shared_ptr< mb_controller > sptr
Definition: mb_controller.hpp:33
Definition: build_info.hpp:12
Definition: power_reference_iface.hpp:22
std::shared_ptr< noc_block_base > sptr
Definition: noc_block_base.hpp:48
boost::noncopyable noncopyable
Definition: noncopyable.hpp:45