Loading [MathJax]/extensions/tex2jax.js
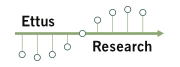 |
USRP Hardware Driver and USRP Manual
Version: 4.8.0.0
UHD and USRP Manual
|
|
Go to the documentation of this file.
167 virtual property<T>& set_coerced(
const T& value) = 0;
177 virtual const T get(
void)
const = 0;
185 virtual const T get_desired(
void)
const = 0;
193 virtual bool empty(
void)
const = 0;
196 template <
typename T>
211 UHD_API std::string leaf(
void)
const;
224 typedef std::shared_ptr<property_tree>
sptr;
231 static sptr make(
void);
234 virtual sptr subtree(
const fs_path& path)
const = 0;
237 virtual void remove(
const fs_path& path) = 0;
240 virtual bool exists(
const fs_path& path)
const = 0;
243 virtual std::vector<std::string> list(
const fs_path& path)
const = 0;
246 template <
typename T>
250 template <
typename T>
254 template <
typename T>
255 std::shared_ptr<property<T>> pop(
const fs_path& path);
259 virtual std::shared_ptr<property_iface> _pop(
const fs_path& path) = 0;
262 virtual void _create(
263 const fs_path& path,
const std::shared_ptr<property_iface>& prop) = 0;
266 virtual std::shared_ptr<property_iface>& _access(
const fs_path& path)
const = 0;
Definition: property_tree.hpp:25
std::function< T(const T &)> coercer_type
Definition: property_tree.hpp:84
#define UHD_API
Definition: config.h:87
std::function< T(void)> publisher_type
Definition: property_tree.hpp:83
std::shared_ptr< property_tree > sptr
Definition: property_tree.hpp:224
UHD_API fs_path operator/(const fs_path &, const fs_path &)
coerce_mode_t
Definition: property_tree.hpp:226
Definition: build_info.hpp:12
Definition: property_tree.hpp:206
Definition: property_tree.hpp:79
Definition: property_tree.hpp:221
std::function< void(const T &)> subscriber_type
Definition: property_tree.hpp:82
virtual ~property(void)=0
Definition: property_tree.hpp:197
boost::noncopyable noncopyable
Definition: noncopyable.hpp:45
#define UHD_API_HEADER
Definition: config.h:88