Loading [MathJax]/extensions/tex2jax.js
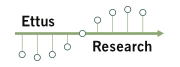 |
USRP Hardware Driver and USRP Manual
Version: 4.8.0.0
UHD and USRP Manual
|
|
Go to the documentation of this file.
14 namespace uhd {
namespace transport {
namespace vrt {
17 static const size_t num_vrl_words32 = 3;
20 static const size_t max_if_hdr_words32 = 7;
41 PACKET_TYPE_DATA = 0x0,
42 PACKET_TYPE_IF_EXT = 0x1,
43 PACKET_TYPE_CONTEXT = 0x2,
48 PACKET_TYPE_ACK = 0x1,
49 PACKET_TYPE_CMD = 0x2,
50 PACKET_TYPE_RESP = 0x3,
173 : link_type(LINK_TYPE_NONE)
174 , packet_type(PACKET_TYPE_DATA)
175 , num_payload_words32(0)
176 , num_payload_bytes(0)
177 , num_header_words32(0)
178 , num_packet_words32(0)
size_t num_header_words32
Definition: vrt_if_packet.hpp:58
uint32_t sid
Definition: vrt_if_packet.hpp:73
link_type_t
Definition: vrt_if_packet.hpp:32
size_t num_payload_words32
Definition: vrt_if_packet.hpp:56
bool error
This is asserted for command responses that are errors (CHDR only)
Definition: vrt_if_packet.hpp:66
bool fc_ack
This is asserted for flow control packets are ACKS (CHDR only)
Definition: vrt_if_packet.hpp:68
uint32_t tsi
Definition: vrt_if_packet.hpp:79
bool has_sid
Stream ID (SID).
Definition: vrt_if_packet.hpp:72
size_t num_payload_bytes
Definition: vrt_if_packet.hpp:57
uint64_t cid
Definition: vrt_if_packet.hpp:76
size_t packet_count
Definition: vrt_if_packet.hpp:62
size_t num_packet_words32
Definition: vrt_if_packet.hpp:59
#define UHD_INLINE
Definition: config.h:65
bool has_tsi
Integer timestamp.
Definition: vrt_if_packet.hpp:78
#define UHD_API
Definition: config.h:87
if_packet_info_t(void)
Definition: vrt_if_packet.hpp:172
bool has_tsf
Fractional timestamp.
Definition: vrt_if_packet.hpp:81
UHD_API void if_hdr_unpack_le(const uint32_t *packet_buff, if_packet_info_t &if_packet_info)
@ error
Definition: log.hpp:121
Definition: build_info.hpp:12
bool has_tlr
Trailer.
Definition: vrt_if_packet.hpp:84
uint64_t tsf
Definition: vrt_if_packet.hpp:82
bool sob
Asserted for start- or end-of-burst.
Definition: vrt_if_packet.hpp:64
UHD_API void if_hdr_pack_be(uint32_t *packet_buff, if_packet_info_t &if_packet_info)
UHD_API void if_hdr_pack_le(uint32_t *packet_buff, if_packet_info_t &if_packet_info)
bool has_cid
Class ID.
Definition: vrt_if_packet.hpp:75
uint32_t tlr
Definition: vrt_if_packet.hpp:85
UHD_API void if_hdr_unpack_be(const uint32_t *packet_buff, if_packet_info_t &if_packet_info)
Definition: vrt_if_packet.hpp:27
packet_type_t
Definition: vrt_if_packet.hpp:39