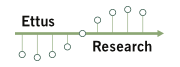 |
USRP Hardware Driver and USRP Manual
Version: 4.4.0.HEAD-0-g5fac246b
UHD and USRP Manual
|
|
Go to the documentation of this file.
23 #include <uhd/version.hpp>
90 struct uhd_rx_streamer;
91 struct uhd_tx_streamer;
134 size_t *num_channels_out
140 size_t *max_num_samps_out
158 size_t samps_per_buff,
214 size_t *num_channels_out
220 size_t *max_num_samps_out
237 size_t samps_per_buff,
387 double *clock_rate_out
407 char* mboard_name_out,
418 int64_t *full_secs_out,
419 double *frac_secs_out
429 int64_t *full_secs_out,
430 double *frac_secs_out
494 const char* time_source,
505 char* time_source_out,
522 const char* clock_source,
533 char* clock_source_out,
567 size_t *num_mboards_out
656 size_t *num_channels_out
663 char* rx_subdev_name_out,
721 UHD_UNUSED(
static const char* UHD_USRP_ALL_LOS) =
"all";
749 char* rx_lo_source_out,
786 double* coerced_freq_out
794 double* rx_lo_freq_out
802 const char *gain_name
829 const char *gain_name,
898 double *bandwidth_out
957 size_t *num_channels_out
964 char* tx_subdev_name_out,
1047 char* tx_lo_source_out,
1048 size_t strbuffer_len
1084 double* coerced_freq_out
1092 double* tx_lo_freq_out
1100 const char *gain_name
1125 const char *gain_name,
1158 size_t strbuffer_len
1179 double *bandwidth_out
UHD_API uhd_error uhd_usrp_get_gpio_attr(uhd_usrp_handle h, const char *bank, const char *attr, size_t mboard, uint32_t *attr_out)
Get a GPIO attribute on a particular GPIO bank.
UHD_API uhd_error uhd_usrp_set_normalized_tx_gain(uhd_usrp_handle h, double gain, size_t chan)
Set the normalized TX gain [0.0, 1.0] for the given channel.
Register info.
Definition: usrp.h:31
UHD_INLINE data_t mask(const soft_reg_field_t field)
Definition: soft_register.hpp:86
UHD_API uhd_error uhd_usrp_get_tx_rates(uhd_usrp_handle h, size_t chan, uhd_meta_range_handle rates_out)
Get a range of possible RX rates for the given channel.
UHD_API uhd_error uhd_usrp_set_clock_source_out(uhd_usrp_handle h, bool enb, size_t mboard)
Enable or disable sending the clock source to an output connector.
UHD_API uhd_error uhd_usrp_get_tx_bandwidth(uhd_usrp_handle h, size_t chan, double *bandwidth_out)
Get the bandwidth for the given channel's TX frontend.
bool stream_now
Stream now?
Definition: usrp.h:83
UHD_API uhd_error uhd_usrp_get_normalized_rx_gain(uhd_usrp_handle h, size_t chan, double *gain_out)
Get the given channel's normalized RX gain [0.0, 1.0].
UHD_API uhd_error uhd_usrp_set_rx_subdev_spec(uhd_usrp_handle h, uhd_subdev_spec_handle subdev_spec, size_t mboard)
Map the given device's RX frontend to a channel.
UHD_API uhd_error uhd_usrp_get_mboard_sensor_names(uhd_usrp_handle h, size_t mboard, uhd_string_vector_handle *mboard_sensor_names_out)
Get a list of motherboard sensors for the given device.
UHD_API uhd_error uhd_rx_streamer_recv(uhd_rx_streamer_handle h, void **buffs, size_t samps_per_buff, uhd_rx_metadata_handle *md, double timeout, bool one_packet, size_t *items_recvd)
Receive buffers containing samples into the given RX streamer.
UHD_API uhd_error uhd_usrp_get_tx_sensor(uhd_usrp_handle h, const char *name, size_t chan, uhd_sensor_value_handle *sensor_value_out)
Get the value for the given TX sensor.
UHD_API uhd_error uhd_usrp_get_time_synchronized(uhd_usrp_handle h, bool *result_out)
Are all motherboard times synchronized?
UHD_API uhd_error uhd_usrp_get_tx_antennas(uhd_usrp_handle h, size_t chan, uhd_string_vector_handle *antennas_out)
Get a list of tx antennas associated with the given channels.
UHD_API uhd_error uhd_usrp_get_gpio_banks(uhd_usrp_handle h, size_t mboard, uhd_string_vector_handle *gpio_banks_out)
Get a list of GPIO banks associated with the given channels.
struct uhd_subdev_spec_t * uhd_subdev_spec_handle
A C-level interface for working with a list of subdevice specifications.
Definition: subdev_spec.h:44
UHD_API uhd_error uhd_usrp_get_master_clock_rate(uhd_usrp_handle h, size_t mboard, double *clock_rate_out)
Get the master clock rate.
@ UHD_STREAM_MODE_START_CONTINUOUS
Stream samples indefinitely.
Definition: usrp.h:64
bool writable
Definition: usrp.h:34
UHD_API uhd_error uhd_usrp_get_rx_subdev_spec(uhd_usrp_handle h, size_t mboard, uhd_subdev_spec_handle subdev_spec_out)
Get the RX frontend specification for the given device.
Define how device streams to host.
Definition: usrp.h:77
UHD_API uhd_error uhd_usrp_get_rx_bandwidth(uhd_usrp_handle h, size_t chan, double *bandwidth_out)
Get the bandwidth for the given channel's RX frontend.
UHD_API uhd_error uhd_usrp_get_rx_info(uhd_usrp_handle h, size_t chan, uhd_usrp_rx_info_t *info_out)
Get RX info from the USRP device.
bool readable
Definition: usrp.h:33
UHD_API uhd_error uhd_usrp_get_rx_rate(uhd_usrp_handle h, size_t chan, double *rate_out)
Get the given RX channel's sample rate (in Sps)
uhd_error
UHD error codes.
Definition: error.h:20
UHD_API uhd_error uhd_usrp_get_rx_lo_names(uhd_usrp_handle h, size_t chan, uhd_string_vector_handle *rx_lo_names_out)
Get a list of possible LO stage names.
UHD_API uhd_error uhd_usrp_set_rx_dc_offset_enabled(uhd_usrp_handle h, bool enb, size_t chan)
Enable or disable RX DC offset correction for the given channel.
UHD_API uhd_error uhd_usrp_get_mboard_name(uhd_usrp_handle h, size_t mboard, char *mboard_name_out, size_t strbuffer_len)
Get the motherboard name for the given device.
UHD_API uhd_error uhd_usrp_set_gpio_attr(uhd_usrp_handle h, const char *bank, const char *attr, uint32_t value, uint32_t mask, size_t mboard)
Set a GPIO attribute for a given GPIO bank.
UHD_API uhd_error uhd_tx_streamer_free(uhd_tx_streamer_handle *h)
Free an TX streamer handle.
UHD_API uhd_error uhd_usrp_set_rx_bandwidth(uhd_usrp_handle h, double bandwidth, size_t chan)
Set the bandwidth for the given channel's RX frontend.
int n_channels
Number of channels.
Definition: usrp.h:55
UHD_API uhd_error uhd_usrp_free(uhd_usrp_handle *h)
Safely destroy the USRP object underlying the handle.
Stores RF and DSP tuned frequencies.
Definition: tune_result.h:18
@ UHD_STREAM_MODE_NUM_SAMPS_AND_DONE
Stream some number of samples and finish.
Definition: usrp.h:68
UHD_API uhd_error uhd_usrp_get_time_last_pps(uhd_usrp_handle h, size_t mboard, int64_t *full_secs_out, double *frac_secs_out)
Get the time when this device's last PPS pulse occurred.
UHD_API uhd_error uhd_usrp_set_dboard_eeprom(uhd_usrp_handle h, uhd_dboard_eeprom_handle db_eeprom, const char *unit, const char *slot, size_t mboard)
Set values in the given daughterboard's EEPROM.
size_t num_samps
Number of samples.
Definition: usrp.h:81
uhd_string_vector_t * uhd_string_vector_handle
Definition: string_vector.h:32
A struct of parameters to construct a stream.
Definition: usrp.h:45
UHD_API uhd_error uhd_rx_streamer_free(uhd_rx_streamer_handle *h)
Free an RX streamer handle.
UHD_API uhd_error uhd_usrp_set_tx_freq(uhd_usrp_handle h, uhd_tune_request_t *tune_request, size_t chan, uhd_tune_result_t *tune_result)
Set the given channel's center TX frequency.
uhd_stream_mode_t
How streaming is issued to the device.
Definition: usrp.h:62
UHD_API uhd_error uhd_usrp_set_rx_agc(uhd_usrp_handle h, bool enable, size_t chan)
Enable or disable the given channel's RX AGC module.
UHD_API uhd_error uhd_usrp_get_tx_freq_range(uhd_usrp_handle h, size_t chan, uhd_meta_range_handle freq_range_out)
Get all possible center frequency ranges for the given channel.
UHD_API uhd_error uhd_usrp_get_tx_stream(uhd_usrp_handle h, uhd_stream_args_t *stream_args, uhd_tx_streamer_handle h_out)
Create TX streamer from a USRP handle and given stream args.
UHD_API uhd_error uhd_usrp_get_dboard_eeprom(uhd_usrp_handle h, uhd_dboard_eeprom_handle db_eeprom, const char *unit, const char *slot, size_t mboard)
Get a handle for the given device's daughterboard EEPROM.
UHD_API uhd_error uhd_usrp_get_rx_sensor_names(uhd_usrp_handle h, size_t chan, uhd_string_vector_handle *sensor_names_out)
Get a list of RX sensors associated with the given channels.
UHD_API uhd_error uhd_usrp_set_tx_bandwidth(uhd_usrp_handle h, double bandwidth, size_t chan)
Set the bandwidth for the given channel's TX frontend.
UHD_API uhd_error uhd_usrp_get_clock_sources(uhd_usrp_handle h, size_t mboard, uhd_string_vector_handle *clock_sources_out)
Get a list of clock sources for the given device.
UHD_API uhd_error uhd_usrp_set_clock_source(uhd_usrp_handle h, const char *clock_source, size_t mboard)
Set the given device's clock source.
UHD_API uhd_error uhd_usrp_set_time_next_pps(uhd_usrp_handle h, int64_t full_secs, double frac_secs, size_t mboard)
Set the USRP device's time to the given value upon the next PPS detection.
size_t * channel_list
Array that lists channels.
Definition: usrp.h:53
UHD_API uhd_error uhd_usrp_get_rx_num_channels(uhd_usrp_handle h, size_t *num_channels_out)
Get the number of RX channels for the given handle.
UHD_API uhd_error uhd_usrp_get_tx_lo_freq(uhd_usrp_handle h, const char *name, size_t chan, double *tx_lo_freq_out)
Get the current Tx LO frequency.
UHD_API uhd_error uhd_usrp_set_tx_rate(uhd_usrp_handle h, double rate, size_t chan)
Set the given RX channel's sample rate (in Sps)
UHD_API uhd_error uhd_usrp_get_tx_lo_names(uhd_usrp_handle h, size_t chan, uhd_string_vector_handle *tx_lo_names_out)
Get a list of possible LO stage names.
#define UHD_API
Definition: config.h:87
UHD_API uhd_error uhd_usrp_get_rx_lo_freq(uhd_usrp_handle h, const char *name, size_t chan, double *rx_lo_freq_out)
Get the current RX LO frequency.
UHD_API uhd_error uhd_usrp_find(const char *args, uhd_string_vector_handle *strings_out)
Find all connected USRP devices.
UHD_API uhd_error uhd_usrp_set_tx_lo_export_enabled(uhd_usrp_handle h, bool enabled, const char *name, size_t chan)
Set whether the LO used by the USRP device is exported.
struct uhd_rx_streamer * uhd_rx_streamer_handle
C-level interface for working with an RX streamer.
Definition: usrp.h:97
USRP TX info.
Definition: usrp_info.h:40
UHD_API uhd_error uhd_usrp_set_time_now(uhd_usrp_handle h, int64_t full_secs, double frac_secs, size_t mboard)
Set the USRP device's time.
uhd_stream_mode_t stream_mode
How streaming is issued to the device.
Definition: usrp.h:79
UHD_API uhd_error uhd_usrp_get_tx_gain_names(uhd_usrp_handle h, size_t chan, uhd_string_vector_handle *gain_names_out)
Get a list of TX gain names for the given channel.
UHD_API uhd_error uhd_tx_streamer_recv_async_msg(uhd_tx_streamer_handle h, uhd_async_metadata_handle *md, double timeout, bool *valid)
Receive an asynchronous message from this streamer.
UHD_API uhd_error uhd_usrp_get_time_sources(uhd_usrp_handle h, size_t mboard, uhd_string_vector_handle *time_sources_out)
Get a list of time sources for the given device.
struct uhd_meta_range_t * uhd_meta_range_handle
C-level interface for dealing with a list of ranges.
Definition: ranges.h:44
UHD_API uhd_error uhd_usrp_get_rx_lo_source(uhd_usrp_handle h, const char *name, size_t chan, char *rx_lo_source_out, size_t strbuffer_len)
Get the currently set LO source.
UHD_API uhd_error uhd_usrp_get_rx_rates(uhd_usrp_handle h, size_t chan, uhd_meta_range_handle rates_out)
Get a range of possible RX rates for the given channel.
UHD_API uhd_error uhd_usrp_get_tx_subdev_name(uhd_usrp_handle h, size_t chan, char *tx_subdev_name_out, size_t strbuffer_len)
Get the name for the RX frontend.
UHD_API uhd_error uhd_usrp_make(uhd_usrp_handle *h, const char *args)
Create a USRP handle.
UHD_API uhd_error uhd_usrp_set_time_source(uhd_usrp_handle h, const char *time_source, size_t mboard)
Set the time source for the given device.
UHD_API uhd_error uhd_usrp_set_user_register(uhd_usrp_handle h, uint8_t addr, uint32_t data, size_t mboard)
Perform a write on a user configuration register bus.
size_t bitwidth
Definition: usrp.h:32
UHD_API uhd_error uhd_usrp_get_rx_gain_range(uhd_usrp_handle h, const char *name, size_t chan, uhd_meta_range_handle gain_range_out)
Get all possible gain ranges for the given channel and name.
UHD_API uhd_error uhd_usrp_get_tx_lo_export_enabled(uhd_usrp_handle h, const char *name, size_t chan, bool *result_out)
Returns true if the currently selected LO is being exported.
struct uhd_usrp * uhd_usrp_handle
C-level interface for working with a USRP device.
Definition: usrp.h:284
UHD_API uhd_error uhd_tx_streamer_max_num_samps(uhd_tx_streamer_handle h, size_t *max_num_samps_out)
Get the max number of samples per buffer per packet.
UHD_API uhd_error uhd_usrp_get_rx_subdev_name(uhd_usrp_handle h, size_t chan, char *rx_subdev_name_out, size_t strbuffer_len)
Get the name for the RX frontend.
@ UHD_STREAM_MODE_NUM_SAMPS_AND_MORE
Stream some number of samples but expect more.
Definition: usrp.h:70
UHD_API uhd_error uhd_usrp_set_tx_lo_freq(uhd_usrp_handle h, double freq, const char *name, size_t chan, double *coerced_freq_out)
Set the Tx LO frequency.
UHD_API uhd_error uhd_usrp_set_rx_rate(uhd_usrp_handle h, double rate, size_t chan)
Set the given RX channel's sample rate (in Sps)
UHD_API uhd_error uhd_usrp_get_tx_num_channels(uhd_usrp_handle h, size_t *num_channels_out)
Get the number of TX channels for the given handle.
UHD_API uhd_error uhd_usrp_set_normalized_rx_gain(uhd_usrp_handle h, double gain, size_t chan)
Set the normalized RX gain [0.0, 1.0] for the given channel.
UHD_API uhd_error uhd_usrp_set_time_source_out(uhd_usrp_handle h, bool enb, size_t mboard)
Enable or disable sending the time source to an output connector.
UHD_API uhd_error uhd_usrp_last_error(uhd_usrp_handle h, char *error_out, size_t strbuffer_len)
Get the last error reported by the USRP handle.
UHD_API uhd_error uhd_rx_streamer_make(uhd_rx_streamer_handle *h)
Create an RX streamer handle.
UHD_API uhd_error uhd_usrp_get_normalized_tx_gain(uhd_usrp_handle h, size_t chan, double *gain_out)
Get the given channel's normalized TX gain [0.0, 1.0].
UHD_API uhd_error uhd_usrp_get_mboard_sensor(uhd_usrp_handle h, const char *name, size_t mboard, uhd_sensor_value_handle *sensor_value_out)
Get the value associated with the given sensor name.
struct uhd_tx_streamer * uhd_tx_streamer_handle
C-level interface for working with a TX streamer.
Definition: usrp.h:103
UHD_API uhd_error uhd_usrp_set_rx_antenna(uhd_usrp_handle h, const char *ant, size_t chan)
Set the RX antenna for the given channel.
UHD_API uhd_error uhd_usrp_get_tx_antenna(uhd_usrp_handle h, size_t chan, char *ant_out, size_t strbuffer_len)
Get the TX antenna for the given channel.
@ UHD_STREAM_MODE_STOP_CONTINUOUS
End continuous streaming.
Definition: usrp.h:66
char * otw_format
Over-the-wire format.
Definition: usrp.h:49
USRP RX info.
Definition: usrp_info.h:17
UHD_API uhd_error uhd_usrp_set_command_time(uhd_usrp_handle h, int64_t full_secs, double frac_secs, size_t mboard)
Set the time at which timed commands will take place.
UHD_API uhd_error uhd_usrp_get_rx_sensor(uhd_usrp_handle h, const char *name, size_t chan, uhd_sensor_value_handle *sensor_value_out)
Get the value for the given RX sensor.
UHD_API uhd_error uhd_usrp_get_rx_gain(uhd_usrp_handle h, size_t chan, const char *gain_name, double *gain_out)
Get the given channel's RX gain.
UHD_API uhd_error uhd_usrp_get_rx_lo_sources(uhd_usrp_handle h, const char *name, size_t chan, uhd_string_vector_handle *rx_lo_sources_out)
Get a list of possible LO sources.
UHD_API uhd_error uhd_usrp_get_tx_lo_source(uhd_usrp_handle h, const char *name, size_t chan, char *tx_lo_source_out, size_t strbuffer_len)
Get the currently set LO source.
UHD_API uhd_error uhd_usrp_set_rx_lo_export_enabled(uhd_usrp_handle h, bool enabled, const char *name, size_t chan)
Set whether the LO used by the USRP device is exported.
UHD_API uhd_error uhd_usrp_set_rx_freq(uhd_usrp_handle h, uhd_tune_request_t *tune_request, size_t chan, uhd_tune_result_t *tune_result)
Set the given channel's center RX frequency.
UHD_API uhd_error uhd_rx_streamer_max_num_samps(uhd_rx_streamer_handle h, size_t *max_num_samps_out)
Get the max number of samples per buffer per packet.
UHD_API uhd_error uhd_tx_streamer_send(uhd_tx_streamer_handle h, const void **buffs, size_t samps_per_buff, uhd_tx_metadata_handle *md, double timeout, size_t *items_sent)
Send buffers containing samples described by the metadata.
UHD_API uhd_error uhd_usrp_get_num_mboards(uhd_usrp_handle h, size_t *num_mboards_out)
Get the number of devices associated with the given USRP handle.
UHD_API uhd_error uhd_usrp_set_tx_lo_source(uhd_usrp_handle h, const char *src, const char *name, size_t chan)
Set the LO source for the USRP device.
UHD_API uhd_error uhd_usrp_clear_command_time(uhd_usrp_handle h, size_t mboard)
Clear the command time so that commands are sent ASAP.
UHD_API uhd_error uhd_usrp_get_rx_gain_names(uhd_usrp_handle h, size_t chan, uhd_string_vector_handle *gain_names_out)
Get a list of RX gain names for the given channel.
char * cpu_format
Format of host memory.
Definition: usrp.h:47
UHD_API uhd_error uhd_usrp_get_rx_freq_range(uhd_usrp_handle h, size_t chan, uhd_meta_range_handle freq_range_out)
Get all possible center frequency ranges for the given channel.
UHD_API uhd_error uhd_usrp_get_rx_bandwidth_range(uhd_usrp_handle h, size_t chan, uhd_meta_range_handle bandwidth_range_out)
Get all possible bandwidth ranges for the given channel's RX frontend.
UHD_API uhd_error uhd_usrp_get_time_now(uhd_usrp_handle h, size_t mboard, int64_t *full_secs_out, double *frac_secs_out)
Get the USRP device's current internal time.
UHD_API uhd_error uhd_usrp_set_rx_lo_source(uhd_usrp_handle h, const char *src, const char *name, size_t chan)
Set the LO source for the USRP device.
UHD_API uhd_error uhd_rx_streamer_num_channels(uhd_rx_streamer_handle h, size_t *num_channels_out)
Get the number of channels associated with this streamer.
UHD_API uhd_error uhd_usrp_get_rx_antennas(uhd_usrp_handle h, size_t chan, uhd_string_vector_handle *antennas_out)
Get a list of RX antennas associated with the given channels.
UHD_API uhd_error uhd_usrp_set_tx_gain(uhd_usrp_handle h, double gain, size_t chan, const char *gain_name)
Set the TX gain for the given channel and name.
UHD_API uhd_error uhd_usrp_get_tx_freq(uhd_usrp_handle h, size_t chan, double *freq_out)
Get the given channel's center TX frequency.
UHD_API uhd_error uhd_usrp_get_pp_string(uhd_usrp_handle h, char *pp_string_out, size_t strbuffer_len)
Get a pretty-print representation of the USRP device.
UHD_API uhd_error uhd_usrp_get_rx_stream(uhd_usrp_handle h, uhd_stream_args_t *stream_args, uhd_rx_streamer_handle h_out)
Create RX streamer from a USRP handle and given stream args.
UHD_API uhd_error uhd_usrp_get_mboard_eeprom(uhd_usrp_handle h, uhd_mboard_eeprom_handle mb_eeprom, size_t mboard)
Get a handle for the given motherboard's EEPROM.
int64_t time_spec_full_secs
If not now, then full seconds into future to stream.
Definition: usrp.h:85
UHD_API uhd_error uhd_usrp_get_tx_gain_range(uhd_usrp_handle h, const char *name, size_t chan, uhd_meta_range_handle gain_range_out)
Get all possible gain ranges for the given channel and name.
UHD_API uhd_error uhd_usrp_get_rx_freq(uhd_usrp_handle h, size_t chan, double *freq_out)
Get the given channel's center RX frequency.
UHD_API uhd_error uhd_usrp_get_tx_gain(uhd_usrp_handle h, size_t chan, const char *gain_name, double *gain_out)
Get the given channel's RX gain.
struct uhd_sensor_value_t * uhd_sensor_value_handle
C-level interface for a UHD sensor.
Definition: sensors.h:35
struct uhd_mboard_eeprom_t * uhd_mboard_eeprom_handle
A C-level interface for interacting with a USRP motherboard's EEPROM.
Definition: mboard_eeprom.h:34
UHD_API uhd_error uhd_usrp_get_tx_info(uhd_usrp_handle h, size_t chan, uhd_usrp_tx_info_t *info_out)
Get TX info from the USRP device.
UHD_API uhd_error uhd_usrp_get_rx_antenna(uhd_usrp_handle h, size_t chan, char *ant_out, size_t strbuffer_len)
Get the RX antenna for the given channel.
UHD_API uhd_error uhd_usrp_get_fe_rx_freq_range(uhd_usrp_handle h, size_t chan, uhd_meta_range_handle freq_range_out)
Get all possible RF frequency ranges for the given channel's RX RF frontend.
UHD_API uhd_error uhd_usrp_get_clock_source(uhd_usrp_handle h, size_t mboard, char *clock_source_out, size_t strbuffer_len)
Get the given device's clock source.
UHD_API uhd_error uhd_rx_streamer_last_error(uhd_rx_streamer_handle h, char *error_out, size_t strbuffer_len)
Get the last error reported by the RX streamer.
UHD_API uhd_error uhd_usrp_get_tx_lo_sources(uhd_usrp_handle h, const char *name, size_t chan, uhd_string_vector_handle *tx_lo_sources_out)
Get a list of possible LO sources.
UHD_API uhd_error uhd_usrp_set_tx_subdev_spec(uhd_usrp_handle h, uhd_subdev_spec_handle subdev_spec, size_t mboard)
Map the given device's TX frontend to a channel.
UHD_API uhd_error uhd_usrp_get_tx_subdev_spec(uhd_usrp_handle h, size_t mboard, uhd_subdev_spec_handle subdev_spec_out)
Get the TX frontend specification for the given device.
UHD_API uhd_error uhd_tx_streamer_make(uhd_tx_streamer_handle *h)
Create an TX streamer handle.
UHD_API uhd_error uhd_usrp_get_rx_lo_export_enabled(uhd_usrp_handle h, const char *name, size_t chan, bool *result_out)
Returns true if the currently selected LO is being exported.
UHD_API uhd_error uhd_usrp_set_time_unknown_pps(uhd_usrp_handle h, int64_t full_secs, double frac_secs)
Synchronize the time across all motherboards.
UHD_API uhd_error uhd_usrp_set_tx_antenna(uhd_usrp_handle h, const char *ant, size_t chan)
Set the TX antenna for the given channel.
UHD_API uhd_error uhd_usrp_get_time_source(uhd_usrp_handle h, size_t mboard, char *time_source_out, size_t strbuffer_len)
Get the time source for the given device.
UHD_API uhd_error uhd_usrp_set_rx_gain(uhd_usrp_handle h, double gain, size_t chan, const char *gain_name)
Set the RX gain for the given channel and name.
UHD_API uhd_error uhd_usrp_get_tx_rate(uhd_usrp_handle h, size_t chan, double *rate_out)
Get the given RX channel's sample rate (in Sps)
UHD_API uhd_error uhd_usrp_set_master_clock_rate(uhd_usrp_handle h, double rate, size_t mboard)
Set the master clock rate.
double time_spec_frac_secs
If not now, then fractional seconds into future to stream.
Definition: usrp.h:87
UHD_API uhd_error uhd_usrp_set_mboard_eeprom(uhd_usrp_handle h, uhd_mboard_eeprom_handle mb_eeprom, size_t mboard)
Set values in the given motherboard's EEPROM.
UHD_API uhd_error uhd_usrp_set_rx_iq_balance_enabled(uhd_usrp_handle h, bool enb, size_t chan)
Enable or disable RX IQ imbalance correction for the given channel.
UHD_API uhd_error uhd_usrp_set_rx_lo_freq(uhd_usrp_handle h, double freq, const char *name, size_t chan, double *coerced_freq_out)
Set the RX LO frequency.
UHD_API uhd_error uhd_usrp_get_fe_tx_freq_range(uhd_usrp_handle h, size_t chan, uhd_meta_range_handle freq_range_out)
Get all possible RF frequency ranges for the given channel's TX RF frontend.
char * args
Other stream args.
Definition: usrp.h:51
UHD_API uhd_error uhd_tx_streamer_last_error(uhd_tx_streamer_handle h, char *error_out, size_t strbuffer_len)
Get the last error reported by the TX streamer.
Instructs implementation how to tune the RF chain.
Definition: tune_request.h:28
UHD_API uhd_error uhd_usrp_get_tx_sensor_names(uhd_usrp_handle h, size_t chan, uhd_string_vector_handle *sensor_names_out)
Get a list of TX sensors associated with the given channels.
UHD_API uhd_error uhd_usrp_get_tx_bandwidth_range(uhd_usrp_handle h, size_t chan, uhd_meta_range_handle bandwidth_range_out)
Get all possible bandwidth ranges for the given channel's TX frontend.
struct uhd_dboard_eeprom_t * uhd_dboard_eeprom_handle
A C-level interface for interacting with a daughterboard EEPROM.
Definition: dboard_eeprom.h:34
UHD_API uhd_error uhd_tx_streamer_num_channels(uhd_tx_streamer_handle h, size_t *num_channels_out)
Get the number of channels associated with this streamer.
UHD_API uhd_error uhd_rx_streamer_issue_stream_cmd(uhd_rx_streamer_handle h, const uhd_stream_cmd_t *stream_cmd)
Issue the given stream command.
UHD_UNUSED(static const char *UHD_USRP_ALL_LOS)
A wildcard for all LO names.