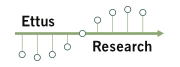 |
USRP Hardware Driver and USRP Manual
Version: 4.8.0.0
UHD and USRP Manual
|
|
Go to the documentation of this file.
12 #include <boost/format.hpp>
13 #include <boost/optional.hpp>
19 namespace uhd {
namespace rfnoc {
namespace chdr {
47 return get_field<uint8_t>(_flat_hdr, VC_OFFSET, VC_WIDTH);
53 _flat_hdr = set_field(_flat_hdr, vc, VC_OFFSET, VC_WIDTH);
59 return get_field<bool>(_flat_hdr, EOB_OFFSET, EOB_WIDTH);
65 _flat_hdr = set_field(_flat_hdr, eob, EOB_OFFSET, EOB_WIDTH);
71 return get_field<bool>(_flat_hdr, EOV_OFFSET, EOV_WIDTH);
77 _flat_hdr = set_field(_flat_hdr, eov, EOV_OFFSET, EOV_WIDTH);
83 return get_field<packet_type_t>(_flat_hdr, PKT_TYPE_OFFSET, PKT_TYPE_WIDTH);
89 _flat_hdr = set_field(_flat_hdr, pkt_type, PKT_TYPE_OFFSET, PKT_TYPE_WIDTH);
95 return get_field<uint8_t>(_flat_hdr, NUM_MDATA_OFFSET, NUM_MDATA_WIDTH);
101 _flat_hdr = set_field(_flat_hdr, num_mdata, NUM_MDATA_OFFSET, NUM_MDATA_WIDTH);
107 return get_field<uint16_t>(_flat_hdr, SEQ_NUM_OFFSET, SEQ_NUM_WIDTH);
113 _flat_hdr = set_field(_flat_hdr, seq_num, SEQ_NUM_OFFSET, SEQ_NUM_WIDTH);
119 return get_field<uint16_t>(_flat_hdr, LENGTH_OFFSET, LENGTH_WIDTH);
125 _flat_hdr = set_field(_flat_hdr, length, LENGTH_OFFSET, LENGTH_WIDTH);
131 return get_field<uint16_t>(_flat_hdr, DST_EPID_OFFSET, DST_EPID_WIDTH);
137 _flat_hdr = set_field(_flat_hdr, dst_epid, DST_EPID_OFFSET, DST_EPID_WIDTH);
147 inline operator uint64_t()
const
155 return _flat_hdr == rhs._flat_hdr;
161 return _flat_hdr != rhs._flat_hdr;
167 _flat_hdr = rhs._flat_hdr;
184 return str(boost::format(
"chdr_header{vc:%u, eob:%c, eov:%c, pkt_type:%u, "
185 "num_mdata:%u, seq_num:%u, length:%u, dst_epid:%u}\n")
194 uint64_t _flat_hdr = 0;
196 static constexpr
size_t VC_WIDTH = 6;
197 static constexpr
size_t EOB_WIDTH = 1;
198 static constexpr
size_t EOV_WIDTH = 1;
199 static constexpr
size_t PKT_TYPE_WIDTH = 3;
200 static constexpr
size_t NUM_MDATA_WIDTH = 5;
201 static constexpr
size_t SEQ_NUM_WIDTH = 16;
202 static constexpr
size_t LENGTH_WIDTH = 16;
203 static constexpr
size_t DST_EPID_WIDTH = 16;
205 static constexpr
size_t VC_OFFSET = 58;
206 static constexpr
size_t EOB_OFFSET = 57;
207 static constexpr
size_t EOV_OFFSET = 56;
208 static constexpr
size_t PKT_TYPE_OFFSET = 53;
209 static constexpr
size_t NUM_MDATA_OFFSET = 48;
210 static constexpr
size_t SEQ_NUM_OFFSET = 32;
211 static constexpr
size_t LENGTH_OFFSET = 16;
212 static constexpr
size_t DST_EPID_OFFSET = 0;
214 static inline uint64_t mask(
const size_t width)
216 return ((uint64_t(1) <<
width) - 1);
219 template <
typename field_t>
220 static inline field_t get_field(
221 const uint64_t flat_hdr,
const size_t offset,
const size_t width)
223 return static_cast<field_t
>((flat_hdr >> offset) & mask(
width));
226 template <
typename field_t>
227 static inline uint64_t set_field(
const uint64_t old_val,
232 return (old_val & ~(mask(
width) << offset))
233 | ((
static_cast<uint64_t
>(field) & mask(
width)) << offset);
269 uint16_t dst_port = 0;
271 uint16_t src_port = 0;
275 boost::optional<uint64_t> timestamp = boost::none;
279 uint16_t src_epid = 0;
281 uint32_t address = 0;
283 std::vector<uint32_t> data_vtr = {0};
285 uint8_t byte_enable = 0xF;
302 size_t serialize(uint64_t* buff,
303 size_t max_size_bytes,
304 const std::function<uint64_t(uint64_t)>& conv_byte_order)
const;
307 template <endianness_t endianness>
308 size_t serialize(uint64_t* buff,
size_t max_size_bytes)
const
310 auto conv_byte_order = [](uint64_t x) -> uint64_t {
312 : uhd::htowx<uint64_t>(x);
314 return serialize(buff, max_size_bytes, conv_byte_order);
321 void deserialize(
const uint64_t* buff,
323 const std::function<uint64_t(uint64_t)>& conv_byte_order);
328 template <endianness_t endianness>
331 auto conv_byte_order = [](uint64_t x) -> uint64_t {
333 : uhd::wtohx<uint64_t>(x);
335 deserialize(buff, buff_size, conv_byte_order);
339 size_t get_length()
const;
344 return bool(timestamp);
353 return !(*
this == rhs);
357 std::string to_string()
const;
360 static constexpr
size_t DST_PORT_WIDTH = 10;
361 static constexpr
size_t SRC_PORT_WIDTH = 10;
362 static constexpr
size_t NUM_DATA_WIDTH = 4;
363 static constexpr
size_t SEQ_NUM_WIDTH = 6;
364 static constexpr
size_t HAS_TIME_WIDTH = 1;
365 static constexpr
size_t IS_ACK_WIDTH = 1;
366 static constexpr
size_t SRC_EPID_WIDTH = 16;
367 static constexpr
size_t ADDRESS_WIDTH = 20;
368 static constexpr
size_t BYTE_ENABLE_WIDTH = 4;
369 static constexpr
size_t OPCODE_WIDTH = 4;
370 static constexpr
size_t STATUS_WIDTH = 2;
373 static constexpr
size_t DST_PORT_OFFSET = 0;
374 static constexpr
size_t SRC_PORT_OFFSET = 10;
375 static constexpr
size_t NUM_DATA_OFFSET = 20;
376 static constexpr
size_t SEQ_NUM_OFFSET = 24;
377 static constexpr
size_t HAS_TIME_OFFSET = 30;
378 static constexpr
size_t IS_ACK_OFFSET = 31;
379 static constexpr
size_t SRC_EPID_OFFSET = 32;
380 static constexpr
size_t ADDRESS_OFFSET = 0;
381 static constexpr
size_t BYTE_ENABLE_OFFSET = 20;
382 static constexpr
size_t OPCODE_OFFSET = 24;
383 static constexpr
size_t STATUS_OFFSET = 30;
384 static constexpr
size_t LO_DATA_OFFSET = 0;
385 static constexpr
size_t HI_DATA_OFFSET = 32;
404 uint16_t src_epid = 0;
408 uint64_t capacity_bytes = 0;
410 uint32_t capacity_pkts = 0;
412 uint64_t xfer_count_bytes = 0;
414 uint64_t xfer_count_pkts = 0;
416 uint16_t buff_info = 0;
418 uint64_t status_info = 0;
431 size_t serialize(uint64_t* buff,
432 size_t max_size_bytes,
433 const std::function<uint64_t(uint64_t)>& conv_byte_order)
const;
436 template <endianness_t endianness>
437 size_t serialize(uint64_t* buff,
size_t max_size_bytes)
const
439 auto conv_byte_order = [](uint64_t x) -> uint64_t {
441 : uhd::htowx<uint64_t>(x);
443 return serialize(buff, max_size_bytes, conv_byte_order);
450 void deserialize(
const uint64_t* buff,
452 const std::function<uint64_t(uint64_t)>& conv_byte_order);
457 template <endianness_t endianness>
460 auto conv_byte_order = [](uint64_t x) -> uint64_t {
462 : uhd::wtohx<uint64_t>(x);
464 deserialize(buff, buff_size, conv_byte_order);
468 size_t get_length()
const;
476 return !(*
this == rhs);
480 std::string to_string()
const;
483 static constexpr
size_t SRC_EPID_WIDTH = 16;
484 static constexpr
size_t STATUS_WIDTH = 4;
485 static constexpr
size_t CAPACITY_BYTES_WIDTH = 40;
486 static constexpr
size_t CAPACITY_PKTS_WIDTH = 24;
487 static constexpr
size_t XFER_COUNT_PKTS_WIDTH = 40;
488 static constexpr
size_t BUFF_INFO_WIDTH = 16;
489 static constexpr
size_t STATUS_INFO_WIDTH = 48;
492 static constexpr
size_t SRC_EPID_OFFSET = 0;
493 static constexpr
size_t STATUS_OFFSET = 16;
494 static constexpr
size_t CAPACITY_BYTES_OFFSET = 24;
495 static constexpr
size_t CAPACITY_PKTS_OFFSET = 0;
496 static constexpr
size_t XFER_COUNT_PKTS_OFFSET = 24;
497 static constexpr
size_t BUFF_INFO_OFFSET = 0;
498 static constexpr
size_t STATUS_INFO_OFFSET = 16;
515 uint16_t src_epid = 0;
521 uint64_t num_pkts = 0;
523 uint64_t num_bytes = 0;
525 static constexpr
size_t MAX_PACKET_SIZE = 128;
538 size_t serialize(uint64_t* buff,
539 size_t max_size_bytes,
540 const std::function<uint64_t(uint64_t)>& conv_byte_order)
const;
543 template <endianness_t endianness>
544 size_t serialize(uint64_t* buff,
size_t max_size_bytes)
const
546 auto conv_byte_order = [](uint64_t x) -> uint64_t {
548 : uhd::htowx<uint64_t>(x);
550 return serialize(buff, max_size_bytes, conv_byte_order);
557 void deserialize(
const uint64_t* buff,
559 const std::function<uint64_t(uint64_t)>& conv_byte_order);
564 template <endianness_t endianness>
567 auto conv_byte_order = [](uint64_t x) -> uint64_t {
569 : uhd::wtohx<uint64_t>(x);
571 deserialize(buff, buff_size, conv_byte_order);
575 size_t get_length()
const;
583 return !(*
this == rhs);
587 std::string to_string()
const;
590 static constexpr
size_t SRC_EPID_WIDTH = 16;
591 static constexpr
size_t OP_CODE_WIDTH = 4;
592 static constexpr
size_t OP_DATA_WIDTH = 4;
593 static constexpr
size_t NUM_PKTS_WIDTH = 40;
596 static constexpr
size_t SRC_EPID_OFFSET = 0;
597 static constexpr
size_t OP_CODE_OFFSET = 16;
598 static constexpr
size_t OP_DATA_OFFSET = 20;
599 static constexpr
size_t NUM_PKTS_OFFSET = 24;
620 MGMT_OP_ADVERTISE = 1,
622 MGMT_OP_SEL_DEST = 2,
626 MGMT_OP_INFO_REQ = 4,
628 MGMT_OP_INFO_RESP = 5,
630 MGMT_OP_CFG_WR_REQ = 6,
632 MGMT_OP_CFG_RD_REQ = 7,
634 MGMT_OP_CFG_RD_RESP = 8
660 cfg_payload(uint16_t addr_, uint32_t data_ = 0) : addr(addr_), data(data_) {}
662 : addr(static_cast<uint16_t>(payload_ >> 0))
663 , data(static_cast<uint32_t>(payload_ >> 16))
684 : device_id(device_id_)
685 , node_type(node_type_)
686 , node_inst(node_inst_)
687 , ext_info(ext_info_)
691 : device_id(static_cast<uint16_t>(payload_ >> 0))
692 , node_type(static_cast<uint8_t>((payload_ >> 16) & 0xF))
693 , node_inst(static_cast<uint16_t>((payload_ >> 20) & 0x3FF))
694 , ext_info(static_cast<uint32_t>((payload_ >> 30) & 0x3FFFF))
699 return ((
static_cast<payload_t>(device_id) << 0)
700 | (
static_cast<payload_t>(node_type & 0xF) << 16)
701 | (
static_cast<payload_t>(node_inst & 0x3FF) << 20)
702 | (
static_cast<payload_t>(ext_info & 0x3FFFF) << 30));
708 const uint8_t ops_pending = 0)
709 : _op_code(op_code), _op_payload(op_payload), _ops_pending(ops_pending)
738 return (_op_code == rhs._op_code) && (_op_payload == rhs._op_payload);
742 std::string to_string()
const;
746 payload_t _op_payload;
747 uint8_t _ops_pending;
781 size_t serialize(std::vector<uint64_t>& target,
782 const std::function<uint64_t(uint64_t)>& conv_byte_order,
783 const size_t padding_size)
const;
788 void deserialize(std::list<uint64_t>& src,
789 const std::function<uint64_t(uint64_t)>& conv_byte_order,
790 const size_t padding_size);
795 return _ops == rhs._ops;
799 std::string to_string()
const;
802 std::vector<mgmt_op_t> _ops;
819 set_src_epid(src_epid);
821 set_proto_ver(protover);
828 _hops.push_back(hop);
846 auto hop = _hops.front();
853 size_t num_lines = 1;
854 for (
const auto& hop : _hops) {
855 num_lines += hop.get_num_ops();
864 size_t serialize(uint64_t* buff,
865 size_t max_size_bytes,
866 const std::function<uint64_t(uint64_t)>& conv_byte_order)
const;
869 template <endianness_t endianness>
870 size_t serialize(uint64_t* buff,
size_t max_size_bytes)
const
872 auto conv_byte_order = [](uint64_t x) -> uint64_t {
874 : uhd::htowx<uint64_t>(x);
876 return serialize(buff, max_size_bytes, conv_byte_order);
883 void deserialize(
const uint64_t* buff,
885 const std::function<uint64_t(uint64_t)>& conv_byte_order);
890 template <endianness_t endianness>
893 auto conv_byte_order = [](uint64_t x) -> uint64_t {
895 : uhd::wtohx<uint64_t>(x);
897 deserialize(buff, buff_size, conv_byte_order);
901 size_t get_length()
const;
904 std::string to_string()
const;
907 std::string hops_to_string()
const;
918 _src_epid = src_epid;
946 _protover = proto_ver;
951 uint16_t _protover = 0;
953 size_t _padding_size = 0;
954 std::deque<mgmt_hop_t> _hops;
958 template <
typename payload_t>
@ CMD_TSERR
Slave asserted a command error.
Definition: chdr_types.hpp:245
@ OP_USER4
Definition: chdr_types.hpp:260
@ OP_SLEEP
Definition: chdr_types.hpp:250
@ OP_READ_WRITE
Definition: chdr_types.hpp:253
Definition: chdr_types.hpp:400
const uint8_t node_type
Definition: chdr_types.hpp:676
size_t get_num_ops() const
Get the number of management operations in this hop.
Definition: chdr_types.hpp:767
constexpr packet_type_t payload_to_packet_type< strc_payload >()
Definition: chdr_types.hpp:974
@ STRC_RESYNC
Trigger a stream status response.
Definition: chdr_types.hpp:508
A class that represents a single management operation.
Definition: chdr_types.hpp:609
size_t serialize(uint64_t *buff, size_t max_size_bytes) const
Serialize the payload to a uint64_t buffer (no conversion function)
Definition: chdr_types.hpp:308
void deserialize(const uint64_t *buff, size_t buff_size)
Definition: chdr_types.hpp:329
size_t serialize(uint64_t *buff, size_t max_size_bytes) const
Serialize the payload to a uint64_t buffer (no conversion function)
Definition: chdr_types.hpp:870
@ PKT_TYPE_CTRL
Stream Command.
Definition: chdr_types.hpp:25
const uint32_t data
Definition: chdr_types.hpp:658
Definition: chdr_types.hpp:265
cfg_payload(payload_t payload_)
Definition: chdr_types.hpp:661
size_t get_num_hops() const
Get the number of management hops in this hop.
Definition: chdr_types.hpp:832
@ OP_USER2
Definition: chdr_types.hpp:258
@ STRC_PING
Initialize stream.
Definition: chdr_types.hpp:507
constexpr size_t chdr_w_to_bits(chdr_w_t chdr_w)
Conversion from chdr_w_t to a number of bits.
Definition: rfnoc_types.hpp:22
const uint16_t addr
Definition: chdr_types.hpp:657
@ CHDR_W_64
Definition: rfnoc_types.hpp:19
const uint16_t device_id
Definition: chdr_types.hpp:675
bool operator==(const mgmt_hop_t &rhs) const
Comparison operator (==)
Definition: chdr_types.hpp:793
@ OP_USER3
Definition: chdr_types.hpp:259
@ STRS_RTERR
Data integrity check failed.
Definition: chdr_types.hpp:397
bool has_timestamp() const
Definition: chdr_types.hpp:342
void set_src_epid(sep_id_t src_epid)
Set the source EPID for this transaction.
Definition: chdr_types.hpp:916
const uint32_t ext_info
Definition: chdr_types.hpp:678
op_code_t
Definition: chdr_types.hpp:616
void set_proto_ver(uint16_t proto_ver)
Set the protocol version for this transaction.
Definition: chdr_types.hpp:944
@ ENDIANNESS_BIG
Definition: endianness.hpp:34
const uint16_t dest
Definition: chdr_types.hpp:643
void add_op(const mgmt_op_t &op)
Add a management operation to this hop.
Definition: chdr_types.hpp:761
UHD_API bool operator==(const time_spec_t &, const time_spec_t &)
Implement equality_comparable interface.
void add_hop(const mgmt_hop_t &hop)
Add a management hop to this transaction.
Definition: chdr_types.hpp:826
#define UHD_API
Definition: config.h:87
@ STRS_OKAY
Definition: chdr_types.hpp:393
const uint16_t node_inst
Definition: chdr_types.hpp:677
bool operator==(const mgmt_op_t &rhs) const
Comparison operator (==)
Definition: chdr_types.hpp:736
mgmt_op_t(const op_code_t op_code, const payload_t op_payload=0, const uint8_t ops_pending=0)
Definition: chdr_types.hpp:706
void deserialize(const uint64_t *buff, size_t buff_size)
Definition: chdr_types.hpp:565
size_t serialize(uint64_t *buff, size_t max_size_bytes) const
Serialize the payload to a uint64_t buffer (no conversion function)
Definition: chdr_types.hpp:544
void deserialize(const uint64_t *buff, size_t buff_size)
Definition: chdr_types.hpp:458
uint16_t sep_id_t
Stream Endpoint ID Type.
Definition: rfnoc_types.hpp:73
@ CMD_WARNING
Slave asserted a time stamp error.
Definition: chdr_types.hpp:246
uint64_t get_op_payload() const
Get the payload for this transaction.
Definition: chdr_types.hpp:730
strc_op_code_t
Definition: chdr_types.hpp:505
@ PKT_TYPE_MGMT
Definition: chdr_types.hpp:22
sel_dest_payload(payload_t payload_)
Definition: chdr_types.hpp:646
@ STRS_CMDERR
No error.
Definition: chdr_types.hpp:394
Definition: chdr_types.hpp:511
constexpr packet_type_t payload_to_packet_type()
Conversion from payload_t to pkt_type.
@ OP_BLOCK_WRITE
Definition: chdr_types.hpp:254
@ OP_POLL
Definition: chdr_types.hpp:256
constexpr packet_type_t payload_to_packet_type< ctrl_payload >()
Definition: chdr_types.hpp:962
@ OP_WRITE
Definition: chdr_types.hpp:251
strs_status_t
Definition: chdr_types.hpp:392
@ PKT_TYPE_DATA_WITH_TS
Data Packet without TimeStamp.
Definition: chdr_types.hpp:27
@ STRS_DATAERR
Packet out of sequence (sequence error)
Definition: chdr_types.hpp:396
ctrl_opcode_t
Definition: chdr_types.hpp:249
Definition: build_info.hpp:12
@ CMD_CMDERR
Transaction successful.
Definition: chdr_types.hpp:244
An interpretation class for the payload for MGMT_OP_INFO_RESP.
Definition: chdr_types.hpp:673
constexpr packet_type_t payload_to_packet_type< mgmt_payload >()
Definition: chdr_types.hpp:968
packet_type_t
Definition: chdr_types.hpp:21
uint64_t payload_t
The payload for an operation is 48 bits wide.
Definition: chdr_types.hpp:638
op_code_t get_op_code() const
Get the op-code for this transaction.
Definition: chdr_types.hpp:724
A class that represents a single management hop.
Definition: chdr_types.hpp:753
Definition: chdr_types.hpp:655
bool operator!=(const strs_payload &rhs) const
Comparison operator (!=)
Definition: chdr_types.hpp:474
void set_header(sep_id_t src_epid, uint16_t protover, chdr_w_t chdr_w)
Definition: chdr_types.hpp:817
@ STRC_INIT
Definition: chdr_types.hpp:506
@ PKT_TYPE_STRC
Stream status.
Definition: chdr_types.hpp:24
bool operator!=(const ctrl_payload &rhs) const
Comparison operator (!=)
Definition: chdr_types.hpp:351
@ OP_USER1
Definition: chdr_types.hpp:257
chdr_w_t get_chdr_w() const
Return the CHDR_W for this transaction.
Definition: chdr_types.hpp:925
@ STRS_SEQERR
A stream command signalled an error.
Definition: chdr_types.hpp:395
uint16_t get_proto_ver() const
Return the protocol version for this transaction.
Definition: chdr_types.hpp:938
void set_chdr_w(chdr_w_t chdr_w)
Set the CHDR_W for this transaction.
Definition: chdr_types.hpp:931
@ PKT_TYPE_DATA_NO_TS
Control Transaction.
Definition: chdr_types.hpp:26
@ OP_USER6
Definition: chdr_types.hpp:262
size_t serialize(uint64_t *buff, size_t max_size_bytes) const
Serialize the payload to a uint64_t buffer (no conversion function)
Definition: chdr_types.hpp:437
bool operator!=(const strc_payload &rhs) const
Comparison operator (!=)
Definition: chdr_types.hpp:581
ctrl_status_t
Definition: chdr_types.hpp:242
sel_dest_payload(uint16_t dest_)
Definition: chdr_types.hpp:645
@ OP_USER5
Definition: chdr_types.hpp:261
const mgmt_hop_t & get_hop(size_t i) const
Get the n'th hop in the transaction.
Definition: chdr_types.hpp:838
@ OP_READ
Definition: chdr_types.hpp:252
node_info_payload(payload_t payload_)
Definition: chdr_types.hpp:690
A class that represents a complete multi-hop management transaction.
Definition: chdr_types.hpp:808
size_t get_size_bytes() const
Definition: chdr_types.hpp:851
node_info_payload(uint16_t device_id_, uint8_t node_type_, uint16_t node_inst_, uint32_t ext_info_)
Definition: chdr_types.hpp:680
@ PKT_TYPE_STRS
Management packet.
Definition: chdr_types.hpp:23
void deserialize(const uint64_t *buff, size_t buff_size)
Definition: chdr_types.hpp:891
UHD_INLINE size_t width(const soft_reg_field_t field)
Definition: soft_register.hpp:75
@ CMD_OKAY
Definition: chdr_types.hpp:243
uint8_t get_ops_pending() const
Get the ops pending for this transaction.
Definition: chdr_types.hpp:718
const mgmt_op_t & get_op(size_t i) const
Get the n'th operation in the hop.
Definition: chdr_types.hpp:773
@ OP_BLOCK_READ
Definition: chdr_types.hpp:255
mgmt_hop_t pop_hop()
Pop the first hop of the transaction and return it.
Definition: chdr_types.hpp:844
constexpr packet_type_t payload_to_packet_type< strs_payload >()
Definition: chdr_types.hpp:980
An interpretation class for the payload for MGMT_OP_SEL_DEST.
Definition: chdr_types.hpp:641
cfg_payload(uint16_t addr_, uint32_t data_=0)
Definition: chdr_types.hpp:660
chdr_w_t
Type that indicates the CHDR Width in bits.
Definition: rfnoc_types.hpp:19
sep_id_t get_src_epid() const
Return the source EPID for this transaction.
Definition: chdr_types.hpp:910