Loading [MathJax]/extensions/tex2jax.js
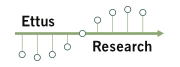 |
USRP Hardware Driver and USRP Manual
Version: 4.8.0.0
UHD and USRP Manual
|
|
Go to the documentation of this file.
12 #include <boost/operators.hpp>
18 namespace uhd {
namespace convert {
24 typedef std::shared_ptr<converter>
sptr;
37 (*this)(in, out, num);
49 virtual void operator()(
66 std::string to_pp_string(
void)
const;
67 std::string to_string(
void)
const;
std::function< converter::sptr(void)> function_type
Conversion factory function typedef.
Definition: convert.hpp:54
int priority_type
Priority of conversion routines.
Definition: convert.hpp:57
Identify a conversion routine in the registry.
Definition: convert.hpp:60
UHD_API function_type get_converter(const id_type &id, const priority_type prio=-1)
UHD_API void register_bytes_per_item(const std::string &format, const size_t size)
std::string input_format
Definition: convert.hpp:62
virtual void set_scalar(const double)=0
Set the scale factor (used in floating point conversions)
size_t num_inputs
Definition: convert.hpp:63
uhd::ref_vector< void * > output_type
Definition: convert.hpp:25
UHD_INLINE void conv(const input_type &in, const output_type &out, const size_t num)
The public conversion method to convert inputs -> outputs.
Definition: convert.hpp:34
#define UHD_INLINE
Definition: config.h:65
#define UHD_API
Definition: config.h:87
std::string output_format
Definition: convert.hpp:64
virtual ~converter(void)=0
Definition: build_info.hpp:12
A conversion class that implements a conversion from inputs -> outputs.
Definition: convert.hpp:21
UHD_API void register_converter(const id_type &id, const function_type &fcn, const priority_type prio)
std::shared_ptr< converter > sptr
Definition: convert.hpp:24
UHD_API bool operator==(const id_type &, const id_type &)
Implement equality_comparable interface.
size_t num_outputs
Definition: convert.hpp:65
uhd::ref_vector< const void * > input_type
Definition: convert.hpp:26
Definition: ref_vector.hpp:21
UHD_API size_t get_bytes_per_item(const std::string &format)
Convert an item format to a size in bytes.