Loading [MathJax]/extensions/tex2jax.js
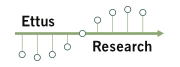 |
USRP Hardware Driver and USRP Manual
Version: 4.8.0.0
UHD and USRP Manual
|
|
Go to the documentation of this file.
11 #include <boost/numeric/conversion/bounds.hpp>
12 #include <boost/version.hpp>
14 #if BOOST_VERSION >= 106700
15 # include <boost/integer/common_factor.hpp>
17 namespace _bmint = boost::integer;
19 # include <boost/math/common_factor.hpp>
20 namespace _bmint = boost::math;
32 static const double PI = 3.14159265358979323846;
50 static const float SINGLE_PRECISION_EPSILON = 1.19e-7f;
51 static const double DOUBLE_PRECISION_EPSILON = 2.22e-16;
61 static constexpr
double FREQ_COMPARE_EPSILON = 1e-12;
63 namespace fp_compare {
78 template <
typename float_t>
107 template <
typename float_t>
110 template <
typename float_t>
113 template <
typename float_t>
116 template <
typename float_t>
119 template <
typename float_t>
122 template <
typename float_t>
128 template <
typename float_t>
130 template <
typename float_t>
132 template <
typename float_t>
134 template <
typename float_t>
136 template <
typename float_t>
138 template <
typename float_t>
141 template <
typename float_t>
143 template <
typename float_t>
145 template <
typename float_t>
147 template <
typename float_t>
149 template <
typename float_t>
151 template <
typename float_t>
179 static const float SINGLE_PRECISION_DELTA = 1e-3f;
180 static const double DOUBLE_PRECISION_DELTA = 1e-5;
183 static const double FREQ_COMPARISON_DELTA_HZ = 0.1;
186 namespace fp_compare {
201 template <
typename float_t>
215 template <
typename float_t>
217 template <
typename float_t>
219 template <
typename float_t>
221 template <
typename float_t>
223 template <
typename float_t>
225 template <
typename float_t>
230 template <
typename float_t>
232 template <
typename float_t>
234 template <
typename float_t>
236 template <
typename float_t>
238 template <
typename float_t>
240 template <
typename float_t>
243 template <
typename float_t>
245 template <
typename float_t>
247 template <
typename float_t>
249 template <
typename float_t>
251 template <
typename float_t>
253 template <
typename float_t>
266 return std::pow(10, (dB_val) / 10.0);
271 return 10 * std::log10(val);
276 template <
typename IntegerType>
277 inline IntegerType
lcm(IntegerType x, IntegerType y)
280 return _bmint::lcm<IntegerType>(x, y);
284 template <
typename IntegerType>
285 inline IntegerType
gcd(IntegerType x, IntegerType y)
288 return _bmint::gcd<IntegerType>(x, y);
298 template <
typename T>
303 return (T(0) < x) - (x < T(0));
318 double freq = std::fmod(requested_freq, rate);
319 if (std::abs(freq) > rate / 2.0)
UHD_INLINE bool operator==(fp_compare_delta< float_t > lhs, fp_compare_delta< float_t > rhs)
Definition: fp_compare_delta.ipp:64
UHD_INLINE bool operator>=(fp_compare_delta< float_t > lhs, fp_compare_delta< float_t > rhs)
Definition: fp_compare_delta.ipp:97
IntegerType gcd(IntegerType x, IntegerType y)
Portable version of gcd() across Boost versions.
Definition: math.hpp:285
double lin_to_dB(const double val)
Definition: math.hpp:269
UHD_INLINE bool frequencies_are_equal(double lhs, double rhs)
Definition: math.hpp:258
An alias for fp_compare_epsilon, but with defaults for frequencies.
Definition: math.hpp:155
float_t _epsilon
Definition: math.hpp:89
#define UHD_INLINE
Definition: config.h:65
constexpr int sign(T x)
Returns the sign of x.
Definition: math.hpp:299
float_t _value
Definition: math.hpp:211
UHD_INLINE freq_compare_epsilon(const freq_compare_epsilon ©)
Definition: math.hpp:163
float_t _delta
Definition: math.hpp:212
Definition: build_info.hpp:12
UHD_INLINE bool operator<=(fp_compare_delta< float_t > lhs, fp_compare_delta< float_t > rhs)
Definition: fp_compare_delta.ipp:84
UHD_INLINE bool operator<(fp_compare_delta< float_t > lhs, fp_compare_delta< float_t > rhs)
Definition: fp_compare_delta.ipp:77
IntegerType lcm(IntegerType x, IntegerType y)
Portable version of lcm() across Boost versions.
Definition: math.hpp:277
UHD_INLINE fp_compare_epsilon(float_t value)
UHD_INLINE fp_compare_delta(float_t value)
UHD_INLINE freq_compare_epsilon(double value)
Definition: math.hpp:158
double wrap_frequency(const double requested_freq, const double rate)
Return a wrapped frequency that is the equivalent frequency in the first.
Definition: math.hpp:316
UHD_INLINE bool operator>(fp_compare_delta< float_t > lhs, fp_compare_delta< float_t > rhs)
Definition: fp_compare_delta.ipp:90
float_t _value
Definition: math.hpp:88
UHD_INLINE void operator=(const fp_compare_epsilon ©)
Definition: fp_compare_epsilon.ipp:51
UHD_INLINE void operator=(const fp_compare_delta ©)
Definition: fp_compare_delta.ipp:56
UHD_INLINE ~fp_compare_epsilon()
Definition: fp_compare_epsilon.ipp:46
UHD_INLINE ~fp_compare_delta()
Definition: fp_compare_delta.ipp:51
UHD_INLINE bool operator!=(fp_compare_delta< float_t > lhs, fp_compare_delta< float_t > rhs)
Definition: fp_compare_delta.ipp:71
double dB_to_lin(const double dB_val)
Definition: math.hpp:264