Loading [MathJax]/extensions/tex2jax.js
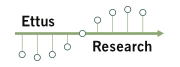 |
USRP Hardware Driver and USRP Manual
Version: 4.8.0.0
UHD and USRP Manual
|
|
Go to the documentation of this file.
41 const std::string& utrue,
42 const std::string& ufalse);
53 const std::string& unit,
54 const std::string& formatter =
"%d");
65 const std::string& unit,
66 const std::string& formatter =
"%f");
75 const std::string& name,
const std::string& value,
const std::string& unit);
85 sensor_value_t(
const std::map<std::string, std::string>& sensor_dict);
94 bool to_bool(
void)
const;
97 signed to_int(
void)
const;
100 double to_real(
void)
const;
123 enum data_type_t { BOOLEAN =
'b', INTEGER =
'i', REALNUM =
'r', STRING =
's' };
129 std::string to_pp_string(
void)
const;
std::string unit
Definition: sensors.hpp:120
data_type_t type
The data type of the value.
Definition: sensors.hpp:126
std::string name
The name of the sensor value.
Definition: sensors.hpp:106
data_type_t
Enumeration of possible data types in a sensor.
Definition: sensors.hpp:123
#define UHD_API
Definition: config.h:87
source
Identify the source of calibration data, i.e., where was it stored.
Definition: database.hpp:22
std::string value
Definition: sensors.hpp:113
Definition: build_info.hpp:12
std::map< std::string, std::string > sensor_map_t
Definition: sensors.hpp:30
Definition: sensors.hpp:28