Loading [MathJax]/extensions/tex2jax.js
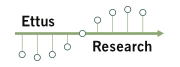 |
USRP Hardware Driver and USRP Manual
Version: 4.8.0.0
UHD and USRP Manual
|
|
Go to the documentation of this file.
20 struct async_metadata_t;
29 typedef std::shared_ptr<device>
sptr;
44 static void register_device(
45 const find_t& find,
const make_t& make,
const device_filter_t filter);
74 const device_addr_t& hint, device_filter_t filter = ANY,
size_t which = 0);
108 virtual bool recv_async_msg(
115 device_filter_t get_device_type()
const;
std::vector< device_addr_t > device_addrs_t
A typedef for a vector of device addresses.
Definition: device_addr.hpp:94
std::shared_ptr< rx_streamer > sptr
Definition: stream.hpp:172
std::shared_ptr< tx_streamer > sptr
Definition: stream.hpp:278
uhd::property_tree::sptr _tree
Definition: device.hpp:118
#define UHD_API
Definition: config.h:87
std::shared_ptr< property_tree > sptr
Definition: property_tree.hpp:224
Definition: device_addr.hpp:38
std::function< device_addrs_t(const device_addr_t &)> find_t
Definition: device.hpp:30
@ USRP
Definition: device.hpp:34
std::function< sptr(const device_addr_t &)> make_t
Definition: device.hpp:31
Definition: build_info.hpp:12
Definition: stream.hpp:50
std::shared_ptr< device > sptr
Definition: device.hpp:29
boost::noncopyable noncopyable
Definition: noncopyable.hpp:45
device_filter_t _type
Definition: device.hpp:119
device_filter_t
Device type, used as a filter in make.
Definition: device.hpp:34
Definition: device.hpp:26